Slices as arguments in Golang
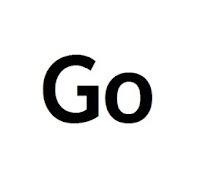
I recently came across several examples of using slices as function arguments, which lead to unexpected results. This post focuses on these examples. First example. The slice is passed to the function as an argument where it will be modified. https://play.golang.org/p/r5mKX5ErwLC package main import "fmt" func change(abc []int) { for i := range abc { abc[i] = 4 } fmt.Println(abc) } func main() { abc := []int{1, 2, 3} change(abc) fmt.Println(abc) } Output: [4 4 4] [4 4 4] Someone might expect that when we pass a slice to a function, we will get a copy of it in the function, and the changes will only appear in the function. But this is not the case. Slices in Go have a base array and are passed by reference, not value. We just pass the slice reference to the function, then change it, and it changes the outer slice. Second example. https://play.golang.org/p/5ruLrp6ZJJc package main import "fmt" func change(abc ...