Can an object access a private member of a class in Java
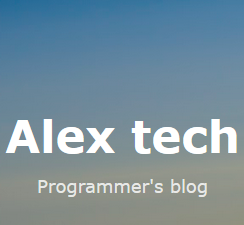
Inside the class, access to a private variable is open without restrictions; The nested class has full access to all (including private) members of the containing class; Access to private variables from the outside can be organized through methods other than private methods provided by the class developer. For example: getX() and setX(). Through the Reflection API: class Example { private int number = 54; } // ... Example example = new Example(); Field field = Example.class.getDeclaredField("number"); field.setAccessible(true); int fieldValue = (int) field.get(example); // ... Read also: What is Application Server How is an abstract class different from an interface in Java Concept of "interface" in Java