What characters can a variable name consist of (a valid identifier) in Java
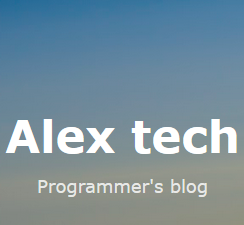
The name or identifier of a variable is a sequence of lowercase and uppercase Latin letters, numbers, as well as the symbols "$" and "_". A variable name can start with any of the listed characters, except a number. It is technically possible to start a variable name with "$" or "_" as well, but this is prohibited by the Java Code Conventions. Also, the dollar symbol "$" is never used at all by convention. By convention, a variable name must begin with a lowercase letter (class names begin with a capital letter). Spaces are not allowed when naming variables. Read also: Methods for reading XML in Java XML, well-formed XML and valid XML XSD